How to add custom fonts to an iOS App
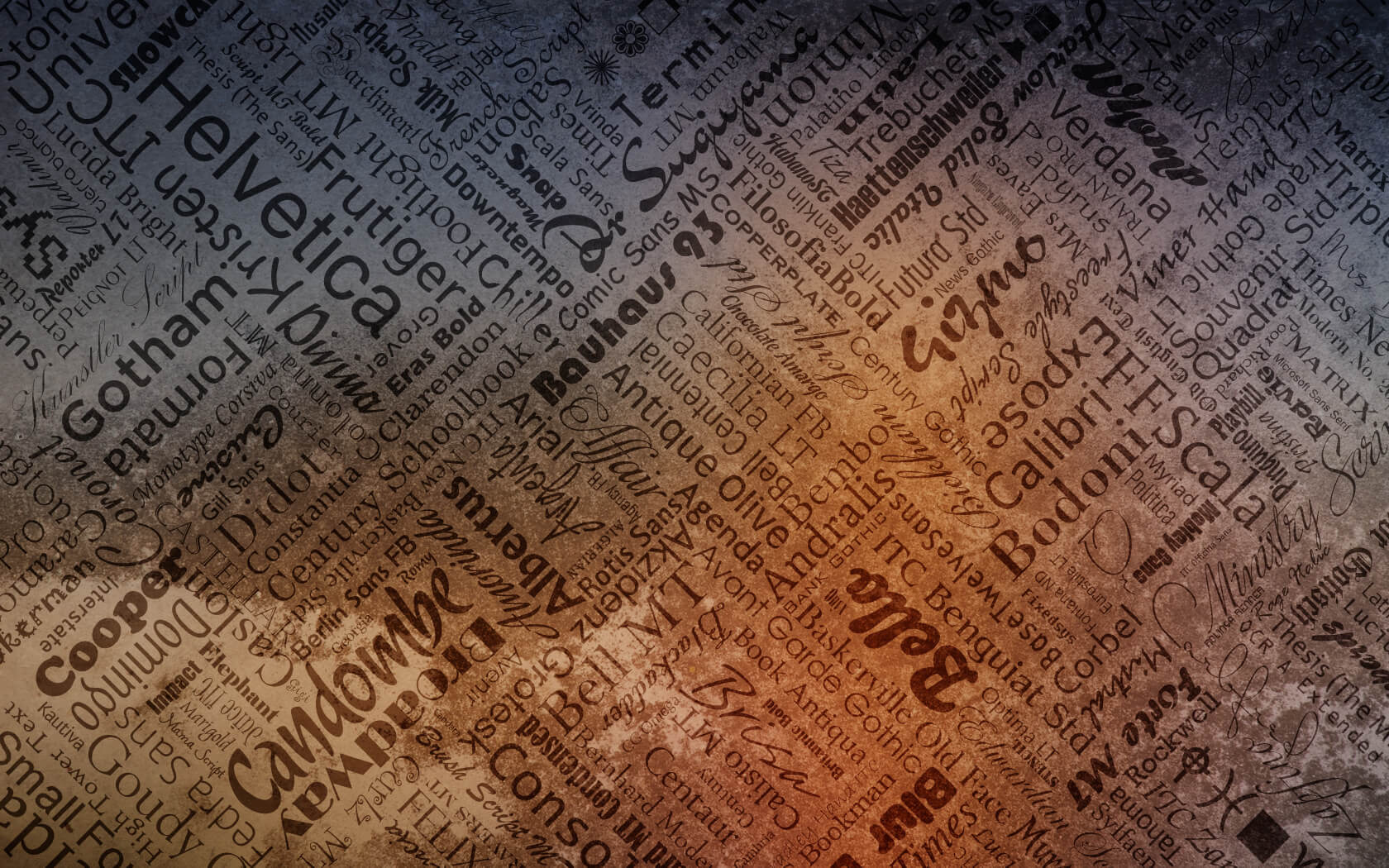
Like colors or images, typography is also an important part of design and can really improve your app. Let’s see how to use Apple supported fonts, but also how to add your own custom font.
1. How to use system fonts
Programmatically
To get font’s real name, you can use the following code. For each font family, it'll list all the font names known by the system.
NSString *family, *font;
for (family in [UIFont familyNames])
{
NSLog(@“\n\tFont Family: %@“, family);
for (font in [UIFont fontNamesForFamilyName:family])
NSLog(@“\t\t Font name: %@\n”, font);
}
You can affect it to your UILabel, UITextview, etc :
UILabel *customLabel = [[UILabel alloc] initWithFrame: CGRectMake(0, 0, 320, 44)];
customLabel.text = @“My perfect font ”;
[customLabel setFont: [UIFont fontWithName:@“AvenirNextCondensed-Regular” size:15]];
[self.view addSubview: customLabel];
2. How to add your own font
Using interface builder
In this example, I am using the font “Gaban font” :
http://www.dafont.com/fr/gaban.font
Step 1 : Include your font to your project
Create a "fonts" folder in "Supporting Files" folder and drag’n’drop your font (ttf or otf) to your folder :
Step 2 : Check your Ressources
Be sure to find your font in your ressource!
Go to your project —> Targets —> Build Phases —> Copy bundle Resources
If your font isn’t there, add it manually by clicking the "+" button. Find your font, select it and click on "add" button :
Step 3 : Include your font in your application plist
In "Supporting Files" folder, open Info.plist file.
By right clicking on the view, add a new row called "Fonts provided by application".
In this row, add your custom font name :
Step 4 : Find the name of your font !
This step is important, because many people just copy/paste the name of their fonts. In fact, the font name may not be what you expect. It could be very different. That’s why going to use the little code we use before to find the system’s font name :
NSString *family, *font;
for (family in [UIFont familyNames])
{
NSLog(@“\n\tFont Family: %@“, family);
for (font in [UIFont fontNamesForFamilyName:family])
NSLog(@“\t\t Font name: %@\n”, font);
}
As you can see, font name is : Gaban
Step 5 : Use your new font !
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(0, 100, self.view.frame.size.width, 150)];
label.textAlignment = NSTextAlignmentCenter;
label.numberOfLines = 2;
label.text = @“My custom font”;
label.font = [UIFont fontWithName:@“Gaban” size:60];
[self.view addSubview:label];
Step 6 : Result
System fonts in iOS 8
Font Family: Marion
Font name: Marion-Italic
Font name: Marion-Bold
Font name: Marion-Regular
Font Family: Copperplate
Font name: Copperplate-Light
Font name: Copperplate
Font name: Copperplate-Bold
Font Family: Heiti SC
Font name: STHeitiSC-Medium
Font name: STHeitiSC-Light
Font Family: Iowan Old Style
Font name: IowanOldStyle-Italic
Font name: IowanOldStyle-Roman
Font name: IowanOldStyle-BoldItalic
Font name: IowanOldStyle-Bold
Font Family: Courier New
Font name: CourierNewPS-BoldMT
Font name: CourierNewPS-ItalicMT
Font name: CourierNewPSMT
Font name: CourierNewPS-BoldItalicMT
Font Family: Apple SD Gothic Neo
Font name: AppleSDGothicNeo-Bold
Font name: AppleSDGothicNeo-Thin
Font name: AppleSDGothicNeo-UltraLight
Font name: AppleSDGothicNeo-Regular
Font name: AppleSDGothicNeo-Light
Font name: AppleSDGothicNeo-Medium
Font name: AppleSDGothicNeo-SemiBold
Font Family: Heiti TC
Font name: STHeitiTC-Medium
Font name: STHeitiTC-Light
Font Family: Gill Sans
Font name: GillSans-Italic
Font name: GillSans-Bold
Font name: GillSans-BoldItalic
Font name: GillSans-LightItalic
Font name: GillSans
Font name: GillSans-Light
Font name: GillSans-SemiBold
Font name: GillSans-SemiBoldItalic
Font name: GillSans-UltraBold
Font Family: Marker Felt
Font name: MarkerFelt-Thin
Font name: MarkerFelt-Wide
Font Family: Thonburi
Font name: Thonburi
Font name: Thonburi-Bold
Font name: Thonburi-Light
Font Family: Avenir Next Condensed
Font name: AvenirNextCondensed-BoldItalic
Font name: AvenirNextCondensed-Heavy
Font name: AvenirNextCondensed-Medium
Font name: AvenirNextCondensed-Regular
Font name: AvenirNextCondensed-HeavyItalic
Font name: AvenirNextCondensed-MediumItalic
Font name: AvenirNextCondensed-Italic
Font name: AvenirNextCondensed-UltraLightItalic
Font name: AvenirNextCondensed-DemiBold
Font name: AvenirNextCondensed-UltraLight
Font name: AvenirNextCondensed-Bold
Font name: AvenirNextCondensed-DemiBoldItalic
Font Family: Tamil Sangam MN
Font name: TamilSangamMN
Font name: TamilSangamMN-Bold
Font Family: Helvetica Neue
Font name: HelveticaNeue-Italic
Font name: HelveticaNeue-Bold
Font name: HelveticaNeue-UltraLight
Font name: HelveticaNeue-CondensedBlack
Font name: HelveticaNeue-BoldItalic
Font name: HelveticaNeue-CondensedBold
Font name: HelveticaNeue-Medium
Font name: HelveticaNeue-Light
Font name: HelveticaNeue-Thin
Font name: HelveticaNeue-ThinItalic
Font name: HelveticaNeue-LightItalic
Font name: HelveticaNeue-UltraLightItalic
Font name: HelveticaNeue-MediumItalic
Font name: HelveticaNeue
Font Family: Gurmukhi MN
Font name: GurmukhiMN-Bold
Font name: GurmukhiMN
Font Family: Times New Roman
Font name: TimesNewRomanPSMT
Font name: TimesNewRomanPS-BoldItalicMT
Font name: TimesNewRomanPS-ItalicMT
Font name: TimesNewRomanPS-BoldMT
Font Family: Georgia
Font name: Georgia-BoldItalic
Font name: Georgia
Font name: Georgia-Italic
Font name: Georgia-Bold
Font Family: Apple Color Emoji
Font name: AppleColorEmoji
Font Family: Arial Rounded MT Bold
Font name: ArialRoundedMTBold
Font Family: Kailasa
Font name: Kailasa-Bold
Font name: Kailasa
Font Family: Kohinoor Devanagari
Font name: KohinoorDevanagari-Light
Font name: KohinoorDevanagari-Medium
Font name: KohinoorDevanagari-Book
Font Family: Sinhala Sangam MN
Font name: SinhalaSangamMN-Bold
Font name: SinhalaSangamMN
Font Family: Chalkboard SE
Font name: ChalkboardSE-Bold
Font name: ChalkboardSE-Light
Font name: ChalkboardSE-Regular
Font Family: Superclarendon
Font name: Superclarendon-Italic
Font name: Superclarendon-Black
Font name: Superclarendon-LightItalic
Font name: Superclarendon-BlackItalic
Font name: Superclarendon-BoldItalic
Font name: Superclarendon-Light
Font name: Superclarendon-Regular
Font name: Superclarendon-Bold
Font Family: Gujarati Sangam MN
Font name: GujaratiSangamMN-Bold
Font name: GujaratiSangamMN
Font Family: Damascus
Font name: DamascusLight
Font name: DamascusBold
Font name: DamascusSemiBold
Font name: DamascusMedium
Font name: Damascus
Font Family: Noteworthy
Font name: Noteworthy-Light
Font name: Noteworthy-Bold
Font Family: Geeza Pro
Font name: GeezaPro
Font name: GeezaPro-Bold
Font Family: Avenir
Font name: Avenir-Medium
Font name: Avenir-HeavyOblique
Font name: Avenir-Book
Font name: Avenir-Light
Font name: Avenir-Roman
Font name: Avenir-BookOblique
Font name: Avenir-Black
Font name: Avenir-MediumOblique
Font name: Avenir-BlackOblique
Font name: Avenir-Heavy
Font name: Avenir-LightOblique
Font name: Avenir-Oblique
Font Family: Academy Engraved LET
Font name: AcademyEngravedLetPlain
Font Family: Mishafi
Font name: DiwanMishafi
Font Family: Futura
Font name: Futura-CondensedMedium
Font name: Futura-CondensedExtraBold
Font name: Futura-Medium
Font name: Futura-MediumItalic
Font Family: Farah
Font name: Farah
Font Family: Kannada Sangam MN
Font name: KannadaSangamMN
Font name: KannadaSangamMN-Bold
Font Family: Arial Hebrew
Font name: ArialHebrew-Bold
Font name: ArialHebrew-Light
Font name: ArialHebrew
Font Family: Arial
Font name: ArialMT
Font name: Arial-BoldItalicMT
Font name: Arial-BoldMT
Font name: Arial-ItalicMT
Font Family: Party LET
Font name: PartyLetPlain
Font Family: Chalkduster
Font name: Chalkduster
Font Family: Hiragino Kaku Gothic ProN
Font name: HiraKakuProN-W6
Font name: HiraKakuProN-W3
Font Family: Hoefler Text
Font name: HoeflerText-Italic
Font name: HoeflerText-Regular
Font name: HoeflerText-Black
Font name: HoeflerText-BlackItalic
Font Family: Optima
Font name: Optima-Regular
Font name: Optima-ExtraBlack
Font name: Optima-BoldItalic
Font name: Optima-Italic
Font name: Optima-Bold
Font Family: Palatino
Font name: Palatino-Bold
Font name: Palatino-Roman
Font name: Palatino-BoldItalic
Font name: Palatino-Italic
Font Family: Malayalam Sangam MN
Font name: MalayalamSangamMN-Bold
Font name: MalayalamSangamMN
Font Family: Lao Sangam MN
Font name: LaoSangamMN
Font Family: Al Nile
Font name: AlNile-Bold
Font name: AlNile
Font Family: Bradley Hand
Font name: BradleyHandITCTT-Bold
Font Family: Hiragino Mincho ProN
Font name: HiraMinProN-W6
Font name: HiraMinProN-W3
Font Family: Trebuchet MS
Font name: Trebuchet-BoldItalic
Font name: TrebuchetMS
Font name: TrebuchetMS-Bold
Font name: TrebuchetMS-Italic
Font Family: Helvetica
Font name: Helvetica-Bold
Font name: Helvetica
Font name: Helvetica-LightOblique
Font name: Helvetica-Oblique
Font name: Helvetica-BoldOblique
Font name: Helvetica-Light
Font Family: Courier
Font name: Courier-BoldOblique
Font name: Courier
Font name: Courier-Bold
Font name: Courier-Oblique
Font Family: Cochin
Font name: Cochin-Bold
Font name: Cochin
Font name: Cochin-Italic
Font name: Cochin-BoldItalic
Font Family: Devanagari Sangam MN
Font name: DevanagariSangamMN
Font name: DevanagariSangamMN-Bold
Font Family: Oriya Sangam MN
Font name: OriyaSangamMN
Font name: OriyaSangamMN-Bold
Font Family: Snell Roundhand
Font name: SnellRoundhand-Bold
Font name: SnellRoundhand
Font name: SnellRoundhand-Black
Font Family: Zapf Dingbats
Font name: ZapfDingbatsITC
Font Family: Bodoni 72
Font name: BodoniSvtyTwoITCTT-Bold
Font name: BodoniSvtyTwoITCTT-Book
Font name: BodoniSvtyTwoITCTT-BookIta
Font Family: Verdana
Font name: Verdana-Italic
Font name: Verdana-BoldItalic
Font name: Verdana
Font name: Verdana-Bold
Font Family: American Typewriter
Font name: AmericanTypewriter-CondensedLight
Font name: AmericanTypewriter
Font name: AmericanTypewriter-CondensedBold
Font name: AmericanTypewriter-Light
Font name: AmericanTypewriter-Bold
Font name: AmericanTypewriter-Condensed
Font Family: Avenir Next
Font name: AvenirNext-UltraLight
Font name: AvenirNext-UltraLightItalic
Font name: AvenirNext-Bold
Font name: AvenirNext-BoldItalic
Font name: AvenirNext-DemiBold
Font name: AvenirNext-DemiBoldItalic
Font name: AvenirNext-Medium
Font name: AvenirNext-HeavyItalic
Font name: AvenirNext-Heavy
Font name: AvenirNext-Italic
Font name: AvenirNext-Regular
Font name: AvenirNext-MediumItalic
Font Family: Baskerville
Font name: Baskerville-Italic
Font name: Baskerville-SemiBold
Font name: Baskerville-BoldItalic
Font name: Baskerville-SemiBoldItalic
Font name: Baskerville-Bold
Font name: Baskerville
Font Family: Khmer Sangam MN
Font name: KhmerSangamMN
Font Family: Didot
Font name: Didot-Italic
Font name: Didot-Bold
Font name: Didot
Font Family: Savoye LET
Font name: SavoyeLetPlain
Font Family: Bodoni Ornaments
Font name: BodoniOrnamentsITCTT
Font Family: Symbol
Font name: Symbol
Font Family: Menlo
Font name: Menlo-Italic
Font name: Menlo-Bold
Font name: Menlo-Regular
Font name: Menlo-BoldItalic
Font Family: Bodoni 72 Smallcaps
Font name: BodoniSvtyTwoSCITCTT-Book
Font Family: DIN Alternate
Font name: DINAlternate-Bold
Font Family: Papyrus
Font name: Papyrus
Font name: Papyrus-Condensed
Font Family: Euphemia UCAS
Font name: EuphemiaUCAS-Italic
Font name: EuphemiaUCAS
Font name: EuphemiaUCAS-Bold
Font Family: Telugu Sangam MN
Font name: TeluguSangamMN
Font name: TeluguSangamMN-Bold
Font Family: Bangla Sangam MN
Font name: BanglaSangamMN-Bold
Font name: BanglaSangamMN
Font Family: Zapfino
Font name: Zapfino
Font Family: Bodoni 72 Oldstyle
Font name: BodoniSvtyTwoOSITCTT-Book
Font name: BodoniSvtyTwoOSITCTT-Bold
Font name: BodoniSvtyTwoOSITCTT-BookIt
Font Family: DIN Condensed
Font name: DINCondensed-Bold
Image credit
Typography by Taryn is licensed under CC BY 2.0